A number input is a special kind of text input that only accepts numbers. The
type of number it accepts is specified by its NumberInput.inputType
.
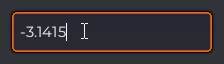
See also:
Static variables
@:value(~/^[\-\+]?[0-9]+(\.[0-9]+)?$/)staticfinalread onlyREG_FLOAT:EReg = ~/^[\-\+]?[0-9]+(\.[0-9]+)?$/
The regular expression used to check whether a string is a valid
signed floating point number. Note that the decimal separator is a
decimal point(.
) as it is common in the English language.
It also accepts strings beginning with a +
or -
sign.
See also:
@:value(~/^[\-\+]?[0-9]+$/)staticfinalread onlyREG_INT:EReg = ~/^[\-\+]?[0-9]+$/
The regular expression used to check whether a string is a valid signed
integer number.
It also accepts strings beginning with a +
or -
sign.
See also:
@:value(~/^[\+?[0-9]+(\.[0-9]+)?$/)staticfinalread onlyREG_UNSIGNED_FLOAT:EReg = ~/^[\+?[0-9]+(\.[0-9]+)?$/
The regular expression used to check whether a string is a valid
unsigned floating point number. Note that the decimal separator is a
decimal point(.
) as it is common in the English language.
It also accepts strings beginning with a +
sign.
See also:
@:value(~/^\+?[0-9]+$/)staticfinalread onlyREG_UNSIGNED_INT:EReg = ~/^\+?[0-9]+$/
The regular expression used to check whether a string is a valid unsigned
integer number.
It also accepts strings beginning with a +
sign.
See also:
Constructor
@:value({ label : "" })new(inputType:NumberType, label:String = "")
Variables
Methods
Return the floating point value of this element. If the input is
invalid, Math.NaN
is returned. This function is equivalent to
getFloatValueOrDefault(Math.NaN)
.
See also:
@:value({ defaultValue : 0.0 })getFloatValueOrDefault(defaultValue:Float = 0.0):Float
Return the floating point value of this element. If the input is
invalid, the given default value is returned.
See also:
Return the integer value of this element. If the input is invalid,
null
is returned (make sure to test this in your code!).
See also:
@:value({ defaultValue : 0 })getIntValueOrDefault(defaultValue:Int = 0):Int
Return the integer value of this element. If the input is invalid, the
given default value is returned.
See also:
Set the floating point value of this element. If this number input
accepts integer vaues only, the decimal places of the given value are
truncated towards zero. If the given value is not a finite number
(Math.NaN
, Math.POSIIVE_INIFINITY
etc.) or null
(possible on
non-static targets only), nothing will happen.
Set the integer value of this element. If the given value is null
(possible on non-static targets only), nothing will happen.
Parameters:
Inherited Variables
@:value("")label:String = ""
The label of this element. It is displayed instead of the text if text
equals ""
.
@:value(Config.textInputMaxLength)maxLength:Int = Config.textInputMaxLength
The maximum length of the text.
See also:
@:value("")read onlytext:String = ""
The current value of this element. To set the text, use
setText()
.
@:value(true)valid:Bool = true
true
if the text value of this TextInput
is valid based on the
regular expression set in validationReg
. If this value is false
, the
text field is highlighted in a different color (theme property
"color_invalid"
).
See also:
A regular expression that checks whether the input text is a valid value
for this text input. If null
, no validation check is performed.
See also:
@:value(Anchor.FollowLayout)anchor:Anchor = Anchor.FollowLayout
The anchor of this element. Used for the layout.
See also:
@:value(false)disabled:Bool = false
true
if the element is disabled and should not react to events. Also,
elements might look different if disabled depending on the theme.
@:value(0)height:Int = 0
The height of this element.
@:value(null)layout:Null<Layout> = null
The layout this element belongs to.
@:value(null)read onlyparent:Null<Element> = null
The parent element of this element.
@:value(0)posX:Int = 0
The x position of this element.
@:value(0)posY:Int = 0
The y position of this element.
@:value(true)visible:Bool = true
If false
, the element is not visible and will not react to any events.
@:value(0)width:Int = 0
The width of this element.
Inherited Methods
Delete the currently selected text.
Return the selected text.
insertText(newText:String, position:Int):Void
Insert the given text at the given position. If the position is greater
or equal than the length of the text, newText
is inserted at the
end of the element's current text. If the new text of this element is
greater than maxLength
, the text is truncated.
Return whether there is some text selected currently.
Return whether the text value of this element is valid according to the
regular expression stored in TextInput.validationReg
.
Select all the text in this text input.
setText(text:String):Void
Set the text of this text input.
finaladdEventListener<T>(eventClass:Class<T>, callback:T ‑> Void):Void
Register a callback to be called if the given event occurs on this
element.
Example:
var button = new Button("Hello!");
button.addEventListener(MouseClickEvent, (event: MouseClickEvent) -> {
if (event.mouseButton == Left && event.getState() == ClickStart) {
trace("Clicked!");
}
});
Parameters:
eventClass | The class of the event, must be a subclass of koui.events.Event |
---|
callback | The callback function to call if the event occurs |
---|
Return the offset of the element's position to the screen.
inlineisAtPosition(x:Int, y:Int):Bool
Return true
if this element overlaps with the given position. Used
internally for event handling most of the time. Elements may override
this method to provide more detailed mouse interaction.
If the element is invisible, false
is returned.
finalinlinelistensTo<T>(eventClass:Class<T>):Bool
Returns whether this element has a registered event listener for the
given event type.
finalinlinelistensToUID<T>(eventTypeUID:String):Bool
Returns whether this element has a registered event listener for the
given event type.
Setup the given element for drawing and call its draw method afterwards.
inlinesetPosition(posX:Int, posY:Int):Void
Set the position of this element. You can also change the position
per axis, see posX
and posY
.
inlinesetSize(width:Int, height:Int):Void
Set the size of this element. You can also change the size for each
individual side, see width
and height
.
setTID(tID:String):Void
Set the theme ID of this element.
Return a string representation of this element:
"Element: <[ClassName]>"